Python Metaclasses
The word “meta” means self-referential. A metaclass is a class whose instances are classes. Like an “ordinary” class defines the
Objects are instances of Classes and the Classes are instances of Metaclasses. We create objects from classes and then we create classes out of metaclasses. In Python, type
is the default metaclass for all the classes but this can be customized as we need.
The term metaprogramming refers to the potential for a program to have knowledge of or manipulate itself. Python supports a form of metaprogramming for classes called metaclasses.
Old-Style vs. New-Style Classes
With old-style classes, class and type are not quite the same instance
obj
obj.__class__
designates the class, type(obj)
instance
New-style classes unify the concepts of class and type. obj
is an instance of a new-style class, type(obj)
is the same as obj.__class__.
Type and Class
In Python 3, all classes are new-style classes. Thus, in Python 3 it is reasonable to refer to an object’s type and its class interchangeably.
In Python 2, classes are old-style by default. Prior to Python 2.2, new-style classes weren’t supported at all. From Python 2.2 onward, they can be created but must be explicitly declared as new-style.
>>> class Foo:
... pass
...
>>> x = Foo()
>>> type(x)
<class '__main__.Foo'>
>>> type(Foo)
<class 'type'>
But the type Foo
type
type
type.
type
is a metaclass, of which classes are instances. Just as an ordinary object is an instance of a class, any new-style class in Python, and thus any class in Python 3, is an instance of the type
metaclass.
In the above case:
x
is an instance ofclass Foo
. Foo
is an instance ofthe type
metaclass .type
is also an instance ofthe type
metaclass , so it is an instance of itself.
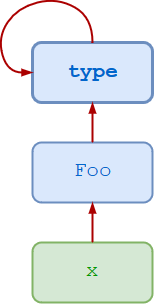
Reference:
https://www.python-course.eu/python3_metaclasses.php
https://realpython.com/python-metaclasses/